How to check if YouTube video is a SHORT
YouTube and Google do not allow to check if a YouTube video is a Short or a long form video in a simple way. Even the YouTube Data V3 does not provide this information. Yes, you can get a video length (ISO8601 formatted duration) and assume that videos shorter then 60 seconds are Shorts. But what if you have a horizontal video of 59 seconds length? A problem.
Luckily, there is a relatively simple way of checking that only if you know the YouTube video ID. Bear in mind, this is an unofficial hack and might stop working without any warning. But when I’m writing those words it works just fine.
The trick is to make a HEAD request to https://www.youtube.com/shorts/{id}
. If you get HTTP response code 303 (See Other) then video is not a Short, but other long for video. HTTP response status 200 (OK) means it is a YouTube Short.
The code that does this check with Apache Http Client looks like this:
package com.quadmeup;
import java.io.IOException;
import java.security.GeneralSecurityException;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpHead;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
public class IsShort {
public static void main(String[] args) throws GeneralSecurityException, IOException, InterruptedException {
try (CloseableHttpClient client = HttpClientBuilder.create().disableRedirectHandling().build()) {
HttpHead request = new HttpHead("https://www.youtube.com/shorts/HnqJKThLEWg");
HttpResponse response = client.execute(request);
if (response.getStatusLine().getStatusCode() == 303) {
System.out.println("Not short");
} else {
System.out.println("Short");
}
}
}
}
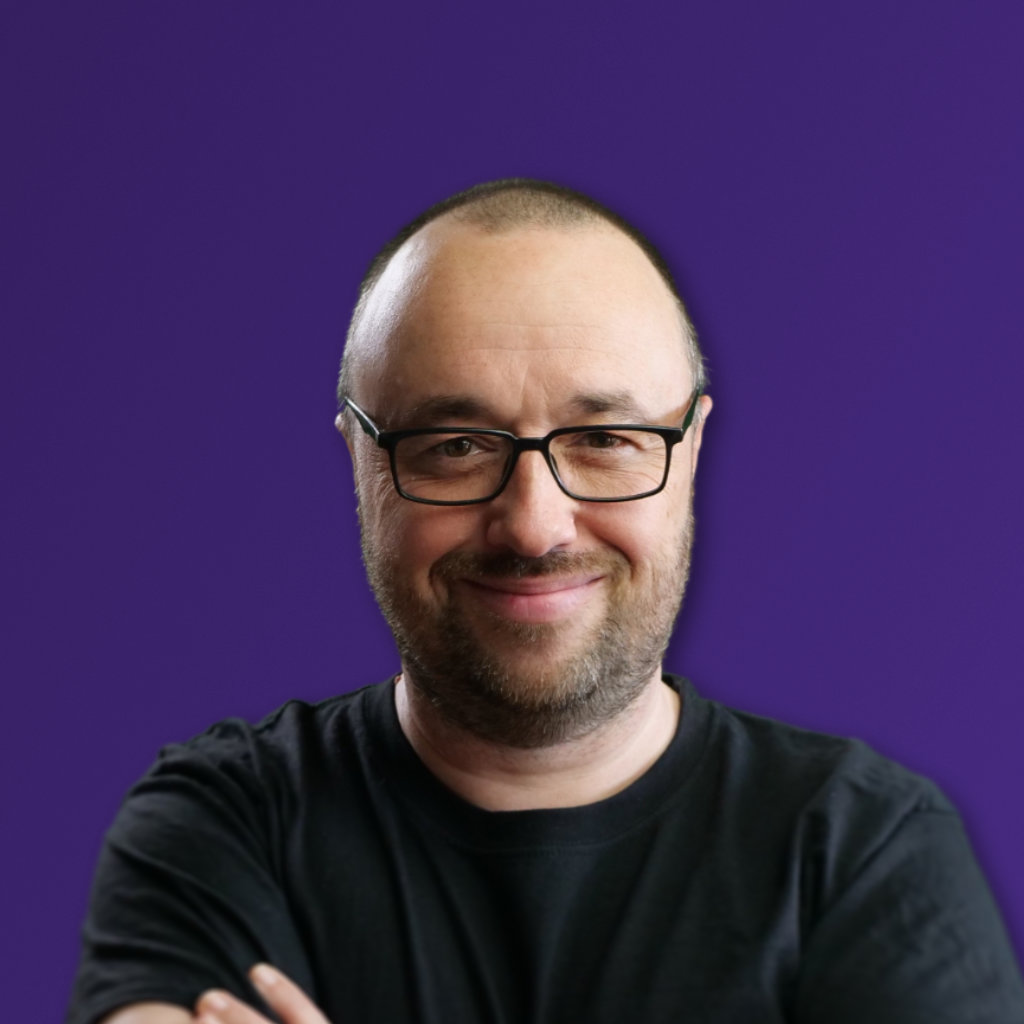
I'm Paweł Spychalski and I do things. Mainly software development, FPV drones and amateur cinematography. Here are my YouTube channels: